piHashVal = (uint64_t*)(result.bResult + 24);
result.bResult is a pointer to some data structure, it's storing the memory address at which the structure begins. We're not interested in the beginning of that data blob, but want to access some 64-bits starting at offset 24. The above expression increments the address by offset 24 and casts the result to pointer to uint64_t. This means that the expression *piHashVal
will return value of those 64-bits, interpreted as unsigned int. Note about the offset, how much bytes it offsets will depend on the type of pointer result.bResult
. See also: Pointer Arithmetic for Structs
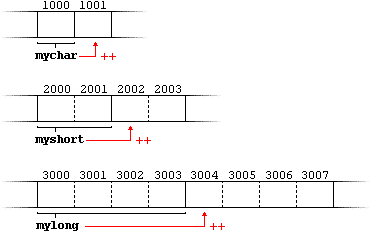
piNonce = (uint32_t*)(oWork.bWorkBlob + 39);
This does similar, only we're taking the 32-bits starting at offset 39 from beginning of oWork.bWorkBlob data structure.
*piNonce = ++result.iNonce;
Increment the result.iNonce
by 1, and assign the resulting value to *piNonce
which is the data stored at memory address piNonce
. Note that both variables result.iNonce
and *piNonce
are changed here. By doing this, we surgically changed some 32-bits in the oWork.BworkBlob
data structure since the piNonce
points to a part of it and we accessed that part directly with *piNonce
.
if (*piHashVal < oWork.iTarget) {
executor::inst()->push_event(ex_event(result, oWork.iPoolId));
}
So here we compare those 64-bits from the start with the target. We're accessing the value *piHashVal
stored at memory location piHashVal
.
Appendix - Pointer Example
#include <stdio.h>
#define ARLEN 2
int main(void)
{
unsigned long a[ARLEN];
unsigned long *b;
unsigned char *c;
for(int i=0; i<ARLEN; ++i)
a[i] = 100 + i*100;
b = &a[0];
/* Note that b = a is equivalent to the above expression, because when a is
* declared as an array it becomes a pointer but the memory block for the array
* is allocated at declaration as well. The [] is also known as offset operator.
* and can be applied to any pointer.
* After the above assignment, a[i] and b[i] will be equivalent.
**/
c = (char*) b;
printf("Accessing using offset operator:\n");
for(int i=0; i<ARLEN; ++i)
printf("a[%d] = %lu = 0x%02X;\n",i,a[i],a[i]);
printf("Accessing using dereference operator:\n");
for(int i=0; i<ARLEN; ++i)
printf("*(b+%d) = %lu = 0x%02X\n",i,*(b+i),*(b+i));
printf("Reading individual bytes of the array using char pointer:\n");
for(int i=0; i < (ARLEN*sizeof(long)/sizeof(char)); ++i)
printf("*(c+%d) = %hhu = 0x%02X;\n",i,*(c+i),*(c+i));
printf("Setting odd bytes to 0xFF:\n");
for(int i=0; i < (ARLEN*sizeof(long)/sizeof(char)); ++i)
{
if (i%2==1)
*(c+i)=0xFF;
printf("*(c+%d) = %hhu = 0x%02X;\n",i,*(c+i),*(c+i));
}
printf("Printing the modified long array:\n");
for(int i=0; i < ARLEN; ++i)
{
printf("a[%d] = %lu = 0x%02X;\n",i,a[i],a[i]);
}
return 0;
}
Program output (compiled on x86-64 system):
Accessing using offset operator:
a[0] = 100 = 0x64;
a[1] = 200 = 0xC8;
Accessing using dereference operator:
*(b+0) = 100 = 0x64
*(b+1) = 200 = 0xC8
Reading individual bytes of the array using char pointer:
*(c+0) = 100 = 0x64;
*(c+1) = 0 = 0x00;
*(c+2) = 0 = 0x00;
*(c+3) = 0 = 0x00;
*(c+4) = 0 = 0x00;
*(c+5) = 0 = 0x00;
*(c+6) = 0 = 0x00;
*(c+7) = 0 = 0x00;
*(c+8) = 200 = 0xC8;
*(c+9) = 0 = 0x00;
*(c+10) = 0 = 0x00;
*(c+11) = 0 = 0x00;
*(c+12) = 0 = 0x00;
*(c+13) = 0 = 0x00;
*(c+14) = 0 = 0x00;
*(c+15) = 0 = 0x00;
Setting odd bytes to 0xFF:
*(c+0) = 100 = 0x64;
*(c+1) = 255 = 0xFF;
*(c+2) = 0 = 0x00;
*(c+3) = 255 = 0xFF;
*(c+4) = 0 = 0x00;
*(c+5) = 255 = 0xFF;
*(c+6) = 0 = 0x00;
*(c+7) = 255 = 0xFF;
*(c+8) = 200 = 0xC8;
*(c+9) = 255 = 0xFF;
*(c+10) = 0 = 0x00;
*(c+11) = 255 = 0xFF;
*(c+12) = 0 = 0x00;
*(c+13) = 255 = 0xFF;
*(c+14) = 0 = 0x00;
*(c+15) = 255 = 0xFF;
Printing the modified long array:
a[0] = 18374966859414962020 = 0xFF00FF64;
a[1] = 18374966859414962120 = 0xFF00FFC8;